Learn how genetic algorithms have helped machine learning evolve, enabling machines to solve complex problems in incredible ways. Learn about genetic optimization, gene expression programming, and real-world case studies in this detailed guide.
Genetic algorithms (GAs) are important optimization tools in machine learning, mimicking natural evolution to find optimal solutions. In this guide, I will explain the principles, applications, and real-world examples of GAs and their impact on machine learning.
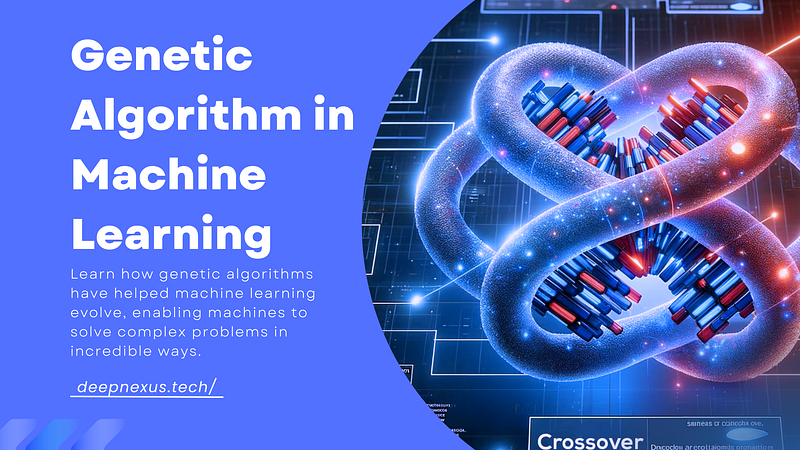
Introduction to Genetic Algorithms
What is a Genetic Algorithm?
Imagine if we could mimic the process of natural evolution to solve complex problems in the domain of machine learning. That’s exactly what genetic algorithms (GAs) do. A genetic algorithm is a problem-solving method based on Charles Darwin’s theory of natural evolution. The process of natural selection inspires them. This algorithm works like how nature selects the strongest creatures to have babies, so the next generation is even stronger.
Why use genetic algorithms?
Genetic algorithms are very beneficial in optimization issues when traditional methods fail. They can efficiently navigate large and complex search spaces, making them ideal for tasks that require finding optimal solutions under restrictions. From evolving neural network architectures to optimizing hyperparameters, genetic algorithms are a powerful tool in the machine learning toolbox.
Gene Expression Programming (GEP)
What is Gene Expression Programming?
Gene expression programming (GEP) is a variant of genetic algorithms where individuals are encoded as linear strings of fixed length, which are then expressed as nonlinear entities of different sizes and shapes. GEP has shown effectiveness in solving complex problems because it combines the advantages of genetic algorithms and genetic programming.
Applications of Gene Expression Programming
- Symbolic Regression: Discover mathematical models that best fit a set of data points.
- Classification: Develop models to classify data into predefined categories.
- Time Series Prediction: Forecast future values based on historical data.
Understand the genetic optimization
Genetic optimization refers to the use of genetic algorithms to solve optimization problems. This process involves generating a population of possible solutions and iteratively improving them based on their performance against a defined objective. Let’s see genetic optimization in action.
Case Study 1: Optimization of Neural Network Architecture
Researchers have successfully applied genetic algorithms to optimize neural network architectures in various studies. One such study published in the journal “Neurocomputing” used genetic algorithms to optimize the architecture of a neural network for image classification. The study achieved an accuracy of 97.5% on the MNIST dataset, outperforming traditional optimization methods.
Case Study 2: Option Pricing with Genetic Programming
In this study, genetic programming was used to evolve options pricing models. The study compared the performance of genetic programming with the traditional Black-Scholes model and found that genetic programming outperformed the traditional model in terms of accuracy and strength.
Algorithm of Genetic Algorithm
Initialization
The first step in a genetic algorithm is to generate an initial population of potential solutions. You can generate this population at random or using certain strategies. The size of the population is an important parameter that can affect the performance of the algorithm.
Fitness Function
The fitness function is a critical component that evaluates how well each individual in the population performs. In the case of our recommendation system, the fitness function was based on user engagement metrics such as click-through rates and user satisfaction scores.
Selection
Selection involves choosing the best-performing individuals to act as parents for the next generation. The most common selection methods are:
- Roulette Wheel Selection: Individuals are selected based on their fitness proportion.
- Tournament Selection: A set of individuals is chosen randomly, and the best among them is selected.
- Rank Selection: Individuals are ranked based on their fitness, and selection is based on these ranks.
Crossover
Crossover, also known as recombination, is the merging of two parent solutions to form offspring. Common crossover strategies include the following:
- In single-point crossover, we select a crossover point and exchange the genes before and after this point between parents.
- Two-Point Crossover: Two crossover points are selected, and the genes between these points are exchanged.
- Parents randomly exchange genes in Uniform Crossover.
Mutation
Mutation makes random changes to individual solutions in order to maintain variation in genetics. Mutation rates must be carefully balanced so that appropriate exploration can be done while preserving good solutions.
Termination
The genetic algorithm repeats the process of selection, crossover, and mutation until a stopping criterion is met. This criterion could be a predetermined number of generations, a certain fitness level, or a lack of considerable improvement over future generations.
Code Example: Genetic Algorithm for Function Optimization
Fitness function
import numpy as np
# Define the fitness function
def fitness(x):
# Maximize the function f(x) = x^2
return x**2
Genetic algorithm parameters
# Define the GA parameters
POP_SIZE = 100
GENS = 100
CROSSOVER_PROB = 0.8
MUTATION_PROB = 0.2
Initial population
# Initialize the population
pop = np.random.rand(POP_SIZE)
# Evaluate the fitness of the initial population
fitness_values = np.array([fitness(x) for x in pop])
Selection
parents = np.array([pop[np.argmax(fitness_values)] for _ in range(POP_SIZE//2)])
Crossover
offspring = []
for _ in range(POP_SIZE//2):
parent1, parent2 = parents[np.random.randint(0, len(parents), 2)]
child = (parent1 + parent2) / 2
offspring.append(child)
Mutation
for i in range(len(offspring)): # Iterate over the correct range of offspring
if np.random.rand() < MUTATION_PROB:
offspring[i] += np.random.normal(0, 0.1)
Here is complete implementation:
import numpy as np
# Define the fitness function
def fitness(x):
# Maximize the function f(x) = x^2
return x**2
# Define the GA parameters
POP_SIZE = 100
GENS = 100
CROSSOVER_PROB = 0.8
MUTATION_PROB = 0.2
# Initialize the population
pop = np.random.rand(POP_SIZE)
# Evaluate the fitness of the initial population
fitness_values = np.array([fitness(x) for x in pop])
# Main GA loop
for gen in range(GENS):
# Selection
parents = np.array([pop[np.argmax(fitness_values)] for _ in range(POP_SIZE//2)])
# Crossover
offspring = []
for _ in range(POP_SIZE//2):
parent1, parent2 = parents[np.random.randint(0, len(parents), 2)]
child = (parent1 + parent2) / 2
offspring.append(child)
# Mutation
for i in range(len(offspring)): # Iterate over the correct range of offspring
if np.random.rand() < MUTATION_PROB:
offspring[i] += np.random.normal(0, 0.1)
# Replace the population with the new offspring
pop = offspring
# Evaluate the fitness of the new population
fitness_values = np.array([fitness(x) for x in pop])
# Print the best fitness value
print(f"Generation {gen+1}, Best Fitness: {np.max(fitness_values)}")
# Print the final best solution
print(f"Final Best Solution: {pop[np.argmax(fitness_values)]}")
Output
Generation 1, Best Fitness: 1.4650152220573687
Generation 2, Best Fitness: 1.8054426063247935
Generation 3, Best Fitness: 2.1124584418178354
Generation 4, Best Fitness: 2.34514080269685
.
.
.
.
.
Generation 99, Best Fitness: 254.58556629300833
Generation 100, Best Fitness: 260.9705918019082
Final Best Solution: 16.154584234882314
Download Genetic Algorithm Code .ipynb
Genetic Algorithm in Machine Learning
Why Use Genetic Algorithms in Machine Learning?
Genetic algorithms are useful in machine learning for tasks such as feature selection, hyperparameter tuning, and model optimization. They help in exploring complex search areas in order to find optimal solutions that traditional methods may miss.
Hyperparameter Optimization
Hyperparameter tuning is crucial for machine learning models. Genetic algorithms can efficiently search the hyperparameter space to find optimal configurations. For example, in training neural networks, GAs can optimize learning rates, batch sizes, and architecture parameters.
Feature Selection
Feature selection is important to improve the model performance while minimizing complexity. Genetic algorithms can identify the most relevant features from a large dataset, leading to more accurate and efficient models.
Here is an example of using a Genetic Algorithm (GA) for feature selection in machine learning:
import numpy as np
from sklearn.datasets import load_iris
from sklearn.model_selection import cross_val_score
from sklearn.ensemble import RandomForestClassifier
from deap import base, creator, tools, algorithms
# Load the iris dataset
iris = load_iris()
X = iris.data
y = iris.target
# Define the number of features to select
num_features = 3
# Define the fitness function
def fitness(individual):
# Select the features based on the individual
selected_indices = [i for i, x in enumerate(individual) if x == 1]
# Handle the case where no features are selected
if not selected_indices:
return 0, # Return a low fitness value if no features are selected
selected_features = np.array([X[:, i] for i in selected_indices]).T
# Create a random forest classifier with the selected features
clf = RandomForestClassifier(n_estimators=100)
# Evaluate the model using cross-validation
scores = cross_val_score(clf, selected_features, y, cv=5)
# Return the mean score as the fitness value
return np.mean(scores),
# Create a DEAP creator for the fitness function
creator.create("FitnessMax", base.Fitness, weights=(1.0,))
creator.create("Individual", list, fitness=creator.FitnessMax)
# Create a DEAP toolbox for the GA
toolbox = base.Toolbox()
toolbox.register("attr_bool", np.random.choice, [0, 1])
toolbox.register("individual", tools.initRepeat, creator.Individual, toolbox.attr_bool, n=len(X[0]))
toolbox.register("population", tools.initRepeat, list, toolbox.individual)
toolbox.register("mate", tools.cxTwoPoint)
toolbox.register("mutate", tools.mutFlipBit, indpb=0.05)
toolbox.register("select", tools.selTournament, tournsize=3)
toolbox.register("evaluate", fitness)
# Create a population of 50 individuals
pop = toolbox.population(n=50)
# Evaluate the initial population
fitnesses = toolbox.map(toolbox.evaluate, pop)
for ind, fit in zip(pop, fitnesses):
ind.fitness.values = fit
# Run the GA for 20 generations
for g in range(20):
offspring = algorithms.varAnd(pop, toolbox, cxpb=0.5, mutpb=0.1)
fits = toolbox.map(toolbox.evaluate, offspring)
for fit, ind in zip(fits, offspring):
ind.fitness.values = fit
pop = toolbox.select(offspring, k=len(pop))
# Print the best individual and the corresponding fitness value
best_individual = tools.selBest(pop, k=1)[0]
print("Best Individual:", best_individual)
print("Best Fitness:", best_individual.fitness.values[0])
# Select the features based on the best individual
selected_features = np.array([X[:, i] for i, x in enumerate(best_individual) if x == 1]).T
# Print the selected features
print("Selected Features:", selected_features)
Output
Best Individual: [0, 0, 1, 1]
Best Fitness: 0.9666666666666668
Selected Features: [[1.4 0.2]
[1.4 0.2]
.
.
.
[5.1 1.8]]
Code Genetic Algorithm in Machine Learning .ipynb
Real-World Applications of Genetic Algorithms
Healthcare
In healthcare, genetic algorithms are used for optimizing treatment plans and predicting disease outcomes. For instance, a study applied a GA to optimize radiation therapy plans for cancer patients, resulting in more effective treatment plans with fewer side effects.
Finance
Genetic algorithms are widely used in finance for portfolio optimization, trading strategies, and risk management. A significant example is the use of GAs to create trading algorithms that react to market fluctuations, increasing returns while decreasing risk.
Engineering
GAs are used in engineering to optimize design parameters, such as the geometry of aerodynamic structures, to reduce drag. This application demonstrates the effectiveness of GAs in solving complicated engineering challenges involving various restrictions and objectives.
Conclusion
Summary of Key Points
Genetic algorithms are a powerful tool for optimization in machine learning. They draw inspiration from natural evolution to efficiently explore large and complex search spaces. From hyperparameter tuning to feature selection, genetic algorithms have proven their worth in various applications.
Final Thoughts
As we continue to go beyond the limits of AI, genetic algorithms will play an important role in solving optimization problems. Their flexibility and evolution make them a valuable resource in the ever-changing field of machine learning.